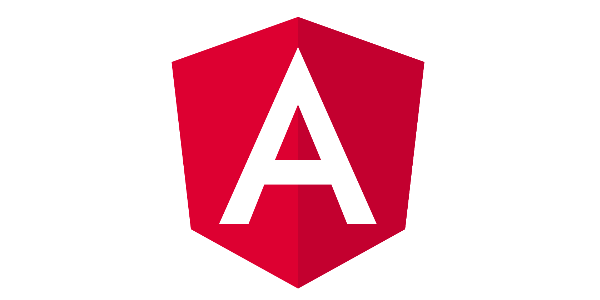
Angular2: method callback type in TypeScript
It's my third post about Angular2 and TypeScript as I'm creating an admin console for OctoPerf load testing platform.
Intro¶
This article explains how to set a type for callbacks. If, like me, you are used to AngularJS you may often use callbacks for asynchronous calls, typically for HTTP requests.
I wrote a StripeClient service that can return the list of my Customers. In pure JavaScript it would be fairly simple as you don't need to give a type to your callback.
In Angular2 / TypeScript¶
In TypeScript it's a bit more complicated, but cleaner.
First I need to define my customer:
export class Customer{
constructor(public email: string, public id:string) {
}
}
Then the method getCustomers of the StripeClient service:
getCustomers(callback: (customers: Customer[])=>void) {
this.http.get(stripeUrl + '/customers', {headers: this.getAuthHeaders()})
.map(res => res.json())
.subscribe(paginatedList => callback(<Customer[]>paginatedList.data));
}
This method uses the Http Service to call the Stripe API. It has only one parameter: the callback function.
The type for the callback is (customers: Customer[])=>void
a method that takes a Customer array as a parameter and returns nothing.
I don't know why be we have to give a name to our customers array.
This method simply calls its callback with the customers returned by the API call.
We need to cast the result when doing so: callback(<Customer[]>paginatedList.data)
.
Stripe returns paginated lists, and we need to make successive calls to their API to get all our customers, plans, etc. It's a good scenario to learn more about generics in TypeScript.