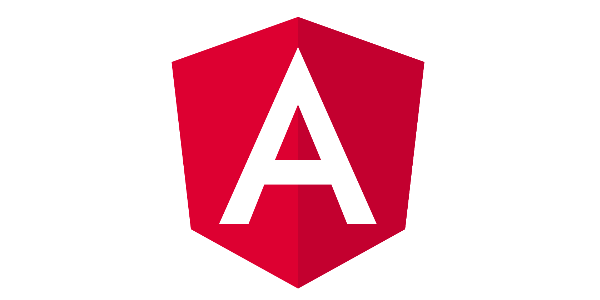
Angular2: simple Drag and Drop
We are using drag and drop extensively for creating virtual users in OctoPerf.
As I plan to migrate to Angular2, today I wanted to check how to handle drag and drop in the future of AngularJS.
I started from the W3Schools plain HTML / JS example and created the following component:
OctoPerf is JMeter on steroids!
Schedule a Demo
import { Component } from 'angular2/core';
@Component({
selector: 'dnd',
directives: [],
styles: [`
.container{
border: 1px solid red;
width: 336px;
height: 69px;
}
`],
template: `
<div id="div1" class="container" (drop)="drop($event)" (dragover)="allowDrop($event)">
<img id="drag1" src="https://www.w3schools.com/html/img_w3slogo.gif" draggable="true"
(dragstart)="drag($event)" width="336" height="69">
</div>
<div id="div2" class="container" (drop)="drop($event)" (dragover)="allowDrop($event)"></div>
`
})
export class DnD {
allowDrop(ev) {
ev.preventDefault();
}
drag(ev) {
ev.dataTransfer.setData("text", ev.target.id);
}
drop(ev) {
ev.preventDefault();
var data = ev.dataTransfer.getData("text");
ev.target.appendChild(document.getElementById(data));
}
}
It's simple as that. The only things that need to be changed are
- The drop methods:
ondrop
becomes(drop)
, - The
event
parameter becomes$event
.
Want to become a super load tester?
Request a Demo