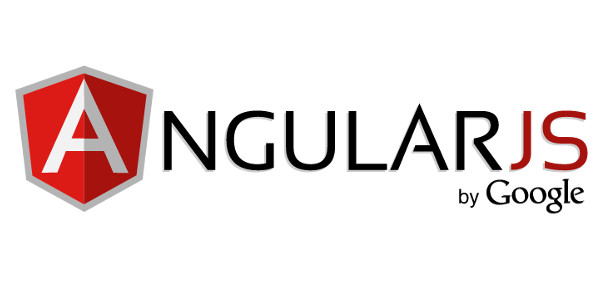
AngularJs domain name filter
Until now, we used default names such as 'Untitled VU' for virtual users created in octoperf, our load testing tool. That's not very relevant. So we changed it to the domain name of the tested website.
The load tester selects a URL to test, and we need to extract the domain name from it.
The Hyperlink Solution¶
A nice trick is to create an hyperlink element <a href="..."/>
. Then we can retrieve the hostname, parsed by the browser.
So instead of relying on a regex we use the browser built-in URI parsing capabilities. It's easier and safer.
Here is the filter code:
app.filter('domain', [ function () {
return function (input) {
var elements = angular.element('<a href="'+input+'"/>');
return elements[0].hostname;
};
}]);
We create a a
element using angular.element
and then retrieve the domain name using elements[0].hostname
. It couldn't be simpler.
To test it, we simply inject the $filter service and check a couple of URLS:
describe('domain filter', function () {
var $filter;
beforeEach(module('app'));
beforeEach(inject(function (_$filter_) {
$filter = _$filter_;
}));
it('should filter urls', function () {
expect($filter('domain')('https://octoperf.com')).toBe('octoperf.com');
expect($filter('domain')('http://blog.octoperf.com')).toBe('blog.octoperf.com');
});
});
Note:
This solution may be less optimized than the regex one. In our case we call this filter only once when a virtual user is created from URLs. So this is not a big deal for us. But to display multiple URLs as hostnames, we'd rather create the
a
element only once (and update its href) or switch back to a regex implementation.