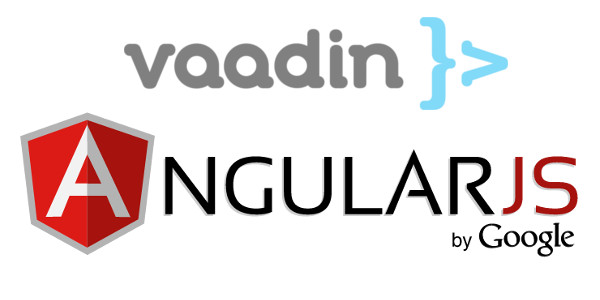
From Java to JavaScript
Our load testing platform, OctoPerf, has its frontend made using AngularJs. But it was not always the case, and this article describes the journey that led us to this choice.
Java was all I knew¶
When I graduated in 2007, all I wanted was to perfect my Java skills. I spent years as a consultant in several companies, chowing down Struts or JSF, Ant and Maven. I also created personal projects to learn JBoss Seam or GWT. Many of these previously trending technologies are outdated now.
Java and its ecosystem were all I knew, and all I wanted to know. The little prick I was, looked at PHP or JS developers with disdain. I was convinced that Java would be the best solution to any problem. I was convinced that I could create web applications without writing HTML or CSS.
You already know that i was wrong. silver bullet is a myth!
Waiting for my computer¶
I often felt bored at the end of my workday. Why so? Because I had spent a large part of it waiting in front of my computer. Waiting for:
- Eclipse to start,
- Ant / Ivy then Maven to download and install dependencies,
- My projects to build, the unit tests to run,
- The Tomcat or JBoss server to start, to deploy my war,
- ...
It was painful each time i had to wait for the whole project to recompile, just because of a small code modification. My boss was paying me to wait. Unbelievable!
[INFO] ------------------------------------------------------------------------
[INFO] BUILD SUCCESS
[INFO] ------------------------------------------------------------------------
[INFO] Total time: 01:15 min
[INFO] Final Memory: 261M/1234M
[INFO] ------------------------------------------------------------------------
I often had that feeling that I had spent my entire day waiting in front of my computer. Even if I had to wait only 30 or 60 seconds per compilation. It was enough for me to switch to other tasks, and most of the time unproductive ones. I must say that it pushed me towards procrastination.
The first step towards the light with PHP¶
Two years ago, I had to write a web application for a demo. A simple CRUD front for a MySQL database. I gave a try to PHP and more specifically to Symfony.
It was the first time I wrote a single line of PHP. I was astonished to have a completely running and stable wep app in under three weeks.
The best part was to instantly see the modifications in my browser. The gain in productivity was humongous. And I could even Unit Test my code! To me, PHP was a dirty language, hard to maintain. I enjoyed being wrong.
Back to old habits, the Vaadin front¶
But when the time came to create OctoPerf, thinking it was the best option to get our product on the market quickly, we first stuck to what we knew best: the Java language.
I read many articles about javascript related technologies, both server side and client side like node.js or AngularJS. This was trendy, and I was curious to know why so many people were using it. There might be a good reason for it?
But some of our team members were more experienced with Vaadin, and we had too much to do with the backend. So we started with Vaadin.
Speaking of the backend, it's entirely written in Java. It is interesting to notice that we tend to make the code more and more concise:
- We massively use Lombok for the data models. It is very handy to create immutable beans, to generate equals, hashcode, toString, getters and setters or even builders. Our java beans are only a few lines of codes long. It integrates pretty well with Spring and made us favor composition over inheritance.
- We switched to Java 8 and enjoy lambda expressions syntax.
The fact is, our backend benefits from an almost 100% test coverage. We use continuous integration extensively. Our entire backend is fully tested in under 15 minutes. So there is no productivity loss due to compile and deploy processes.
The first version of our frontend did not. And the productivity was poor.
- Vaadin requires a mutable data model. So we could not reuse our server code for the front. Worst! We had to duplicate all data models!
- The cross browser compatibility is a joke, especially when we used widgets. We still had to put our hands on JS code to make it all work.
- The GUI is built like in good old Swing, there was no UiBinding like in GWT. I think that it is really easier to correlate your code and the generated GUI using HTML or XML than with plain Java.
- Did I spoke about compilation times?
After a few months, I told myself that we were heading in the wrong direction. We dared to throw away huge parts of our backend code, when it did not comply with our needs. So why not trying something else for the front? AngularJS seemed to be the trend. I gave it a try.
Switching to AngularJS¶
I started with AngularJS documentation and tutorial, to understand the basics of this technology, then quickly switched to a sample application to learn by doing.
The Good¶
The first thing that blew my mind, was the number of graphical themes and widgets available. I'm not a CSS guru and it really eased my job to start from a nice template, based on bootstrap with many widgets to choose from.
The second thing was the gain of productivity. WebStorm starts within seconds. No more Building workspace with my laptop's fan running like crazy for minutes. Open the project, type npm start and you're ready to work. Every code modification can be checked in the browser instantly. Working at HTML level made it really easy for me, a CSS learner, to create a clean and beautiful GUI.
Moreover, the concepts used in AngularJs are common: I already knew factories, services, controllers and dependency injections from my java experience. So I felt quite at home on this part.
angular.module('app', [
'ngAnimate',
'ngCookies',
'ngResource',
'ngSanitize',
'ngStorage'
]);
The Bad¶
But build process is harder to comprehend. I'm not saying that Maven is easy to understand, but at least there is only one tool to learn. The npm / bower / grunt stack was a big mess to me when I started. Hopefully there is a large community of users and many plugins.
The SonarQube integration is a bit tricky, and Unit Tests are harder to maintain than in Java. Even so WebStorm can handle some code refactoring, it is not as efficient. Auto-completion is not really helpful, and I tend not to really use it. I was a CTRL+ SPACE maniac on Eclipse. This is not the case anymore. That said, I really like the structure of the unit test cases with karma. You really write them like you would write your product specifications. However, i'm not a Test Driven Development addict and still write my unit tests after the controllers or services.
There are only two things that hurt my productivity:
- Dependency injection and code minification: you have to write the name of the dependency twice, that's annoying.
- Directives: these drove me nuts. I still have to read the documentation to achieve writing a directive isolate scope properly. How am I supposed to remember the meaning of '&', '=', 'A', 'E' or 'C'? It feels more like computer readable code than human readable code to me! And why the heck would a variable declared as 'onClose' in my scope should be called with on-close in my HTML code. I lost too much time on these. Even worst, it is quite hard to unit test them, and I still have to do it for all my directives.
angular.module('directivesTest', [])
.directive('myCustomer', function() {
return {
restrict: 'E',
scope: {
customerInfo: '=info'
},
templateUrl: 'my-customer-iso.html'
};
});
And the Pretty¶
After about 3 months, we add a first functional version of the frontend. It takes a few minutes to write the resources that would call our server REST endpoints. Writing clean and nice HTML / CSS is not that hard using Bootstrap and Less CSS pre-processor.
We modified the complete build to obfuscate Javascript, CSS and HTML, and to handle the bower dependencies: our index.html file is generated by a simple grunt build dev command and includes all JS and CSS references. It make it really easy to add any widget.
We also had to refactor our controllers when we learnt about Controller As syntax. Nevertheless, the productivity with AngularJS is awesome. There was no Java framework I knew about that would let me create such a complete and modern GUI in such a short time.
AngularJS made it easy for me to switch to JavaScript. You can debug your code in the browser, and see modifications by pressing CTRL + F5. Even so GWT has its Super Dev Mode, I'm still really comfortable with Angular.
What Next? Angular 2!¶
Learning something new took us time. But as the tool is more adapted to our needs, we saved a lot in the end. Learning new things is an investment that always pays for itself in the long run. In fact our need for continuous learning is one the reasons that made us create OctoPerf.
So I'm not going to relax now. AngularJS is quite good, but not perfect. What could improve my developer experience of it? I truly hope that Angular 2 will! I will switch to it only for the directives refactoring.
And if TypeScript is quick to compile (lets say no more than 5 seconds for my whole project), if it generates human readable code, and if IDEs provide powerful autocompletion, this is going to kick ass. A POC on Angular 2 and TypeScript is the next step for our frontend.