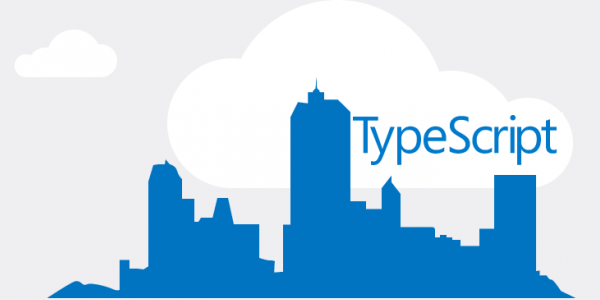
TypeScript using WebStorm
We use AngularJs at OctoPerf.com, for the frontend of our load testing tool. As AngularJs V2 quick start guide uses TypeScript, I think it's a good motivation to give it a try.
Prerequisite¶
Node.js and npm must be installed to run this sample. WebStorm is used to automatically transpile our .ts files (compile them to .js ones).
We can also use the command line transpiler:
npm install -g typescript
to install it.tsc --watch -m commonjs greeter.ts
to transpile it.
WebStorm configuration¶
First we need to configure WebStorm:
- In File open the Settings... panel (Ctrl + Alt +S shortcut on Ubuntu),
- In Languages & Frameworks select TypeScript,
- Select the option Enable TypeScript Compiler,
- In Command Line Options: type --module commonjs,
- And set the value dev (or js or whatever you like) for the Use output path: so we don't mix TypeScript and JavaScript files.
Note:
If you're using git you can also add the line
dev/
to your .gitignore file.
The Greeter.ts sample¶
In webstorm, create greeting.ts file:
import http = require("http");
class Greeter{
greet(): string {
return "Hello world";
}
}
class NameGreeter extends Greeter{
name:string;
constructor (name: string){
super();
this.name = name;
}
greet(): string {
return "Hello "+ this.name;
}
}
http.createServer(function(request, response) {
response.writeHead(200, {"Content-Type": "text/plain"});
var greeter:Greeter;
greeter = new Greeter;
response.write(greeter.greet());
response.write(' ... ');
greeter = new NameGreeter('world');
response.write(greeter.greet());
response.end();
}).listen(8888);
The file greeter.js is automatically created by WebStorm:
var __extends = this.__extends || function (d, b) {
for (var p in b) if (b.hasOwnProperty(p)) d[p] = b[p];
function __() { this.constructor = d; }
__.prototype = b.prototype;
d.prototype = new __();
};
var http = require("http");
var Greeter = (function () {
function Greeter() {
}
Greeter.prototype.greet = function () {
return "Hello world";
};
return Greeter;
})();
var NameGreeter = (function (_super) {
__extends(NameGreeter, _super);
function NameGreeter(name) {
_super.call(this);
this.name = name;
}
NameGreeter.prototype.greet = function () {
return "Hello " + this.name;
};
return NameGreeter;
})(Greeter);
http.createServer(function (request, response) {
response.writeHead(200, { "Content-Type": "text/plain" });
var greeter;
greeter = new Greeter;
response.write(greeter.greet());
response.write(' ... ');
greeter = new NameGreeter('world');
response.write(greeter.greet());
response.end();
}).listen(8888);
//# sourceMappingURL=greeter.js.map
You can check the TypeScript Console to view the transpiler errors and status.
Running the sample code¶
To run the sample code simply execute the command node dev/greeter.js
.
You can then browse http://localhost:8888 to view the result.
It should display Hello world ... Hello world
.
Note:
When we modify the greeter.ts file, it is automatically converted to dev/greeter.js. But node.js does not refresh by default.
If you don't want to manually restart node after each modification, you can use nodemon:
sudo npm install -g nodemon
. Then runnodemon dev/greeter.js
instead.
Conclusion¶
I kept getting a transpile error in my WebStorm console: Error:(1, 23) TS2307: Cannot find external module 'http'.
Even if everything went fine even with this error, I should investigate on how to help TypeScript handle external modules.
This is a very basic TypeScript sample, there are still many things to try:
- Interfaces,
- Generics,
- Mixins,
- Etc.